Python and machine learning is fast becoming a fundamental skill for acoustic and DSP engineers.
While learning Python, there are some very basic terms that you need to understand in order to comprehend the basics of the Python programming language and how it works.
The term “object” is fundamental to python, which is an object-orientated programming language.
In Python, everything is an object, and every object belongs to a class. Attributes, methods, lists, tuples, dictionary, and everything else with data, is an object. We can only really understand objects alongside classes, as classes decide what will happen to an object.
At first, that might be very difficult to understand. I had big problems trying to grasp this when I first started learning python, so let’s break it down further and see what an object is in terms of python code structure.
Below is a visual image of what an object actually is in python.
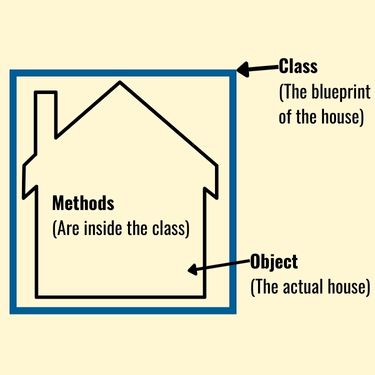
Here is a very simple way to understand what an object is in the world of python:
- A Class is like the blueprint of a house.
- An Object is the actual house itself.
- A Method (known as a function in other programming languages) actually sits inside of the class.
Let’s take the following piece of code as an example to demonstrate what an object actually is.
The following code will check if a student is over the age of 18.
class StudentAge: # This is my class which will check if a student is over 18 years of age.
def check_student_age(self): # This is a method, and is within the class.
if self.age >= 18:
return True
else:
return False
def __init__(self, name, age):
self.name = name
self.age = age
# To run this code, we need to input attributes, which is the age of the students.
student1 = StudentAge("Dave", 23)
student2 = StudentAge("Sarah", 16)
student3 = StudentAge("Tim", 27)
age_check = student1.check_student_age()
print(age_check)
age_check = student2.check_student_age()
print(age_check)
age_check = student3.check_student_age()
print(age_check)
The objects in this piece of code are the students.
It is never good to have attributes (known as variables in other coding languages) within a class itself, as this does not make the class reusable.
This is where Python offers a very elegant solution in the form of the __init__ method.
To explain the above code in simple terms, here is how it works.
- We have a class called StudentAge
This class can be called upon to “do something”, which is done via the two internal methods:
def check_student_age(self)
def __init__(self, name, age) - The method, def check_student_age(self), will look at the age of a student and tell us if the student is younger or older than the age of 18.
- The method, def __init__(self, name, age), is a very elegant way of assigning the attributes’ input name and age.
The init method is a special method that automatically gets called every time objects are created.
To explain the above code in simple terms, here is how it works.
- In the above code, student1, student2 and student3 are objects.
student1 = StudentAge(“Dave”, 23), will pass the attributes to name and age into the class StudentAge.
What Does Self Mean In Python Code?
Self is used to access attributes within the class. Self can be any word, but it is a convention within Python, so it is best to keep it.
Whenever we define methods of a class, we need to use self as the first argument. “Self” represents the object calling the method.
For example, in the following code:
- self refers to the student1 object
- self.age refers to the age attribute of the student1 object
- self.name refers to the name attribute of the student 1 object
In the following code, the attributes “Dave” and “23” are passed to name and age automatically.
Self represents the object calling it, which is student1 in this case.
class StudentAge: # This is my class which will check if a student is over 18 years of age.
def check_student_age(self): # This is a method, and is within the class.
if self.age >= 18:
return True
else:
return False
def __init__(self, name, age):
self.name = name
self.age = age
# To run this code, we need to input attributes, which is the age of the students.
student1 = StudentAge("Dave", 23)
age_check = student1.check_student_age()
print(age_check)
Final Thoughts
Grasping the concept of objects in python can be very tricky, especially if you are new to the code or if you have come from a non-object programming-orientated background.
The best way to get to terms with the concepts is to have a go at different tasks. Try to write a simple piece of code, passing objects into a class with a method.
With time and practice, the logic will come.
To learn more about what objects are in Python, and if you have the time, I recommend checking out Programiz, which has some excellent examples to follow.